Playing blurred video in background with GPUImage
Updated 02 Aug 2022
2 Min
4811 Views
In one project I had a requirement to implement blurred video playing in background. This video had to be blurred in real time. It couldn't be added to the project already blurred. So I'm going to tell you about my solution.
To perform this task I decided to use GPUImage library. GPUImage is a very powerful framework. It takes advantage of the GPU and lets you apply different effects to images, live camera video, and movies.
Also this video had to be shown on different controllers in navigation controller hierarchy. When user transitioned from one controller to another, the video had to be played in background independently from controller, without any pauses or replays. So I created a subclass of the UINavigationController and added the code for blurring video to it's view. Also I set the background for other controllers in hierarchy which had to display this video to transparent.
Additionally the video had to be overlayed with transparent image with combination of radial and linear gradients to make the video darker with blue hue.
How to implement background video
GPUImage framework was added to project through cocoapods. To make classes of this framework available in code the import directive was used:
#import "GPUImage.h"
Here is the code, placed in -viewDidLoad method of my custom UINavigationController, that applies blur effect to video:
- (void)viewDidLoad {
[super viewDidLoad];
GPUImageView *gpuImageView = [[GPUImageView alloc] initWithFrame:[[UIScreen mainScreen] bounds]];
[self.view insertSubview:gpuImageView atIndex:0];
UIImageView *imageView = [[UIImageView alloc] initWithImage:[UIImage imageNamed:@"grd_blur"]];
imageView.frame = [[UIScreen mainScreen] bounds];
[self.view insertSubview:imageView aboveSubview:gpuImageView];
NSURL *fileURL = [[NSBundle mainBundle] URLForResource:@"steps_video" withExtension:@"mov"];
GPUImageMovie *gpuMovie = [[GPUImageMovie alloc] initWithURL:fileURL];
gpuMovie.playAtActualSpeed = YES;
gpuMovie.shouldRepeat = YES;
GPUImageBoxBlurFilter *filter = [[GPUImageBoxBlurFilter alloc] init];
filter.blurRadiusInPixels = 8;
[gpuMovie addTarget:filter];
[filter addTarget:gpuImageView];
[gpuMovie startProcessing];
}
First, I created GPUImageView object, which is a view that contains the blurred video. Also I created UIImageView object that overlays the blurred video. This UIImageView contains custom transparent image with radial and linear gradients and is placed above GPUImageView. The image file grd_blur.png was added to the project's assets.
Then I created GPUImageMovie object initializing it with URL for the source video. The video file steps_video.mov was added to the project's resources. I set the playAtActualSpeed and shouldRepeat attributes to YES. The first attribute made the video to be played at it's actual speed. The second made the video to be played from beginning when it finished.
Next, I created GPUImageBoxBlurFilter object which performs blurring. The degree of blurring was set with blurRadiusInPixels attribute to 8. I added this filter as a target of the GPUImageMovie and set the GPUImageView as a target of the GPUImageBoxBlurFilter.
What we got as a result
Finally, I called startProcessing on the GPUImageMovie to begin the processing of the video.
In the result we have nice blurred video playing in background:
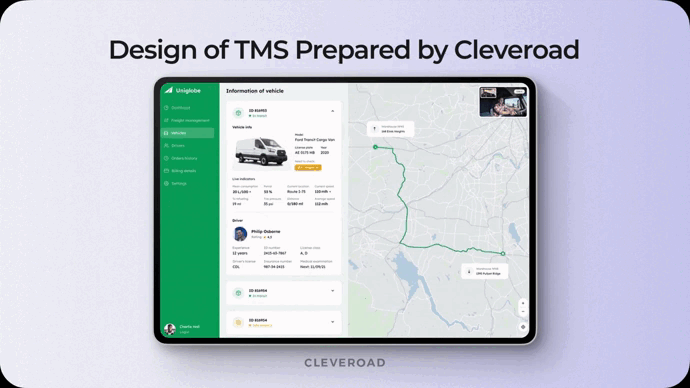
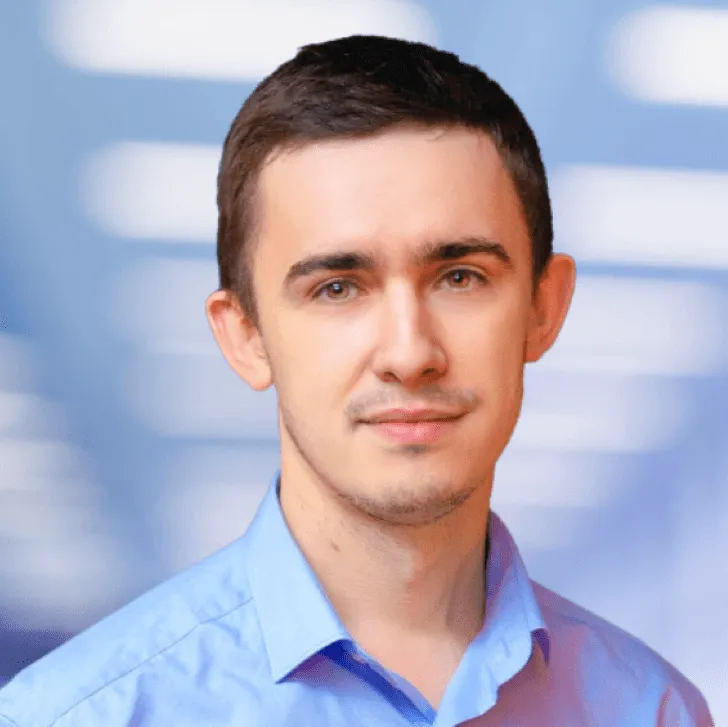
Evgeniy Altynpara is a CTO and member of the Forbes Councils’ community of tech professionals. He is an expert in software development and technological entrepreneurship and has 10+years of experience in digital transformation consulting in Healthcare, FinTech, Supply Chain and Logistics
Give us your impressions about this article
Give us your impressions about this article