SQLite vs Realm: Choosing the Best Database for Android Apps
Updated 07 Jun 2023
10 Min
25534 Views
While developing an Android mobile application you will face a need to store app data somewhere. A database you create can either make the final product perfect or destroy all your hard work. Since there are several options, we are going to help you with the choice and compare two the most popular database solutions for Android development. It's a high time to see who will win a constant battle SQLite vs Realm.
What databases for Android app are there?
When a young Android developer starts looking for the best solution to store data in his first Android mobile app, he can find thousands of ideas. Especially, when he wants to store the data on a server. But if all the information should be stored on a mobile device, there are only a few options. They are SQLite and Realm.
1. SQLite
I would be surprised if you haven't heard anything about SQLite. SQLite is an embedded database designed by Richard Hipp in 2000. It's free and open source. The main idea of SQLite is to get rid of server-client architecture and store all the app information directly on a mobile device. In such a manner, the majority of Android developers have been using it for more than 20 years for the projects where they need to store the data on a device, not on a server.
The DB is written in C and can support more than 30 programming languages. There are thousands of mobile applications which use SQLite in their architecture. Yet, despite all the great features of SQLite DB, it also has a range of drawbacks and imperfections which led to a release of the second most famous and popular DB solutions - Realm.
If you want to code faster and more effectively, check our top ten frameworks for Android development! These tools are really great, we know because we use them every day!
2. Realm
What is Realm database? In simple terms, Realm is a non-relational database management system which allows you to declare relationships between objects the same as you would do in any object graph in your language of choice. Realm is mostly used for mobile app development.
Realm is much younger than SQLite. It was specifically designed to solve all the issues which a young developer face once he or she starts using it. That is why Realm gained such a wide popularity all around the world.
To understand why Realm became so popular and highly demanded, we need to look closer at the general issues which a young developer faces when he or she uses SQLite for the first time.
SQLite problems
Frankly speaking, SQLite isn't that bad for some small projects. And it can fulfil all the requirements of your app if it's not too complex. In all other cases, you'll have to deal with the following difficulties:
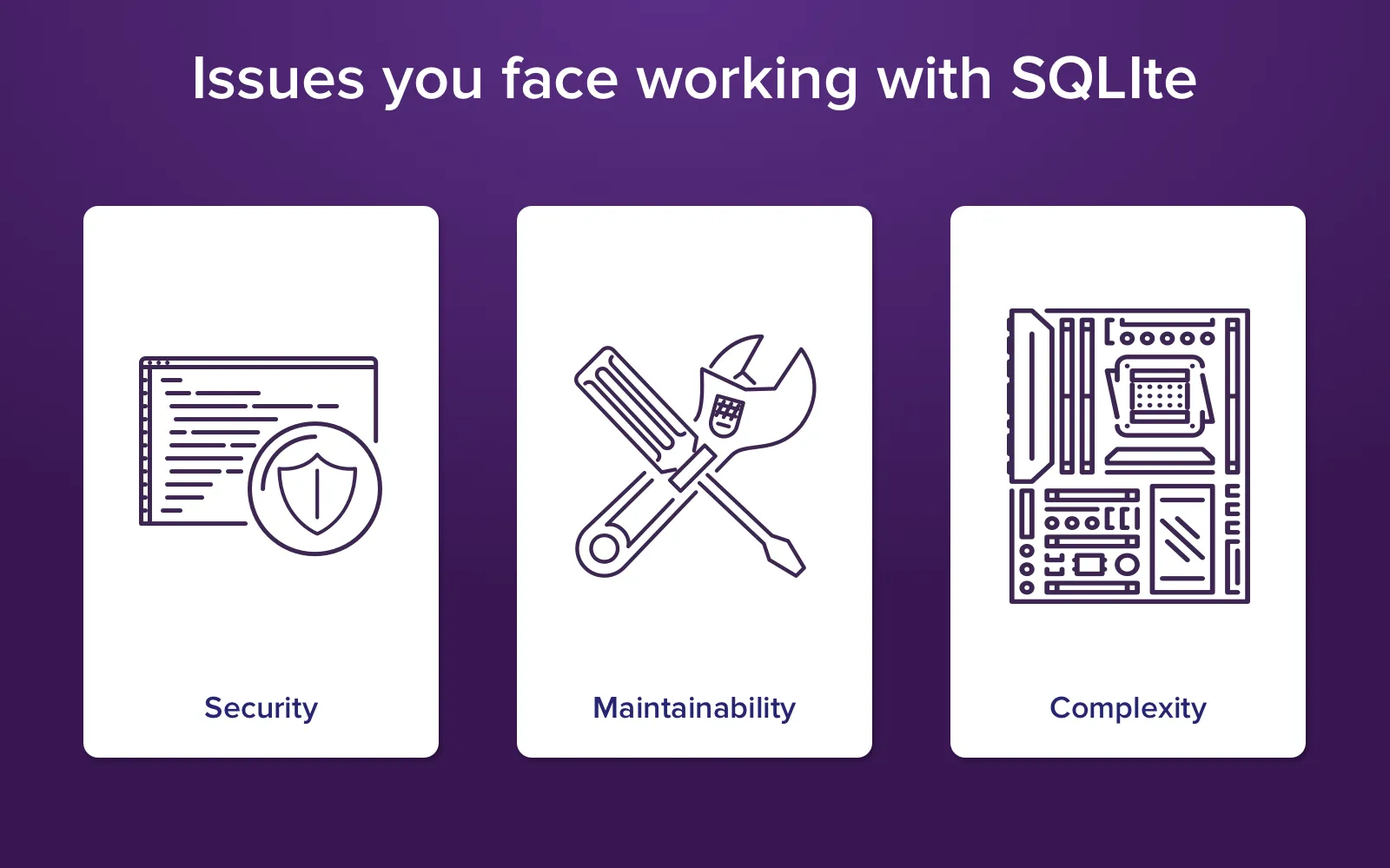
SQLite disadvantages
Security
Due to a specific structure of SQLite and it's peculiarities, there are several security issues which you need to know about before you start using SQLite database in Android apps. First of all, you need to pay attention to a fact that SQLite DB is stored in one file. This file you can put wherever you like in the directory hierarchy. At first glance it may seem to be very convenient, yet, there's a risk that a database can be opened and rewritten by any rogue process. That is why the security should be provided at the level of a file. Be very attentive setting permissions and keep the files out of the web root.
Another SQLite problem concerning security occurs when it comes to journaling. To have a rollback option, the database creates temporary journal file. As a rule, they get deleted after a transaction. Yet, there are some cases when these files aren't deleted. As a result, the data in such a database can be corrupted due to this peculiarity of SQLite database in Android. But don't think that turning journaling off can help. If you do that, the database will be damaged if the app crashes. As an option, you can encrypt your data before putting them into the DB. Besides, SQLite as a special extension for these purposes.
During your Android app development, don't forget about your app security! You can find some great approaches and techniques for your customers' data protection in our short video right here!
Mobile App and Data Security in Android
Maintainability and scalability
It is logical that the database of your app will grow with the time. Along with that, the need to write more complex queries will rise as well. The majority of young programmers face lots of issues during this phase because they have little experience with the structured query language. The same problem you'll face during data migration. Below, we'll give you an example.
Complexity
For experienced mobile developers who have worked with SQL and SQLite for a few years, all these issues are very easy to solve. But for newbies who have just started applying SQLite for Android development, this DB and it's complex massive structure feels like a real headache. That's why the new solution which isn't based on SQL at all and is very simple to work with created a huge hype in the programming sphere when it was released in 2017. We are talking about Realm.
Difference between SQLite and Realm
Once Realm was released thousands of developers felt it's advantages over SQLite. Of course, Cleveroad also tested Realm database in Android development to compare it with the systems which we usually use and find the best solution. Here's what pluses we've noticed:
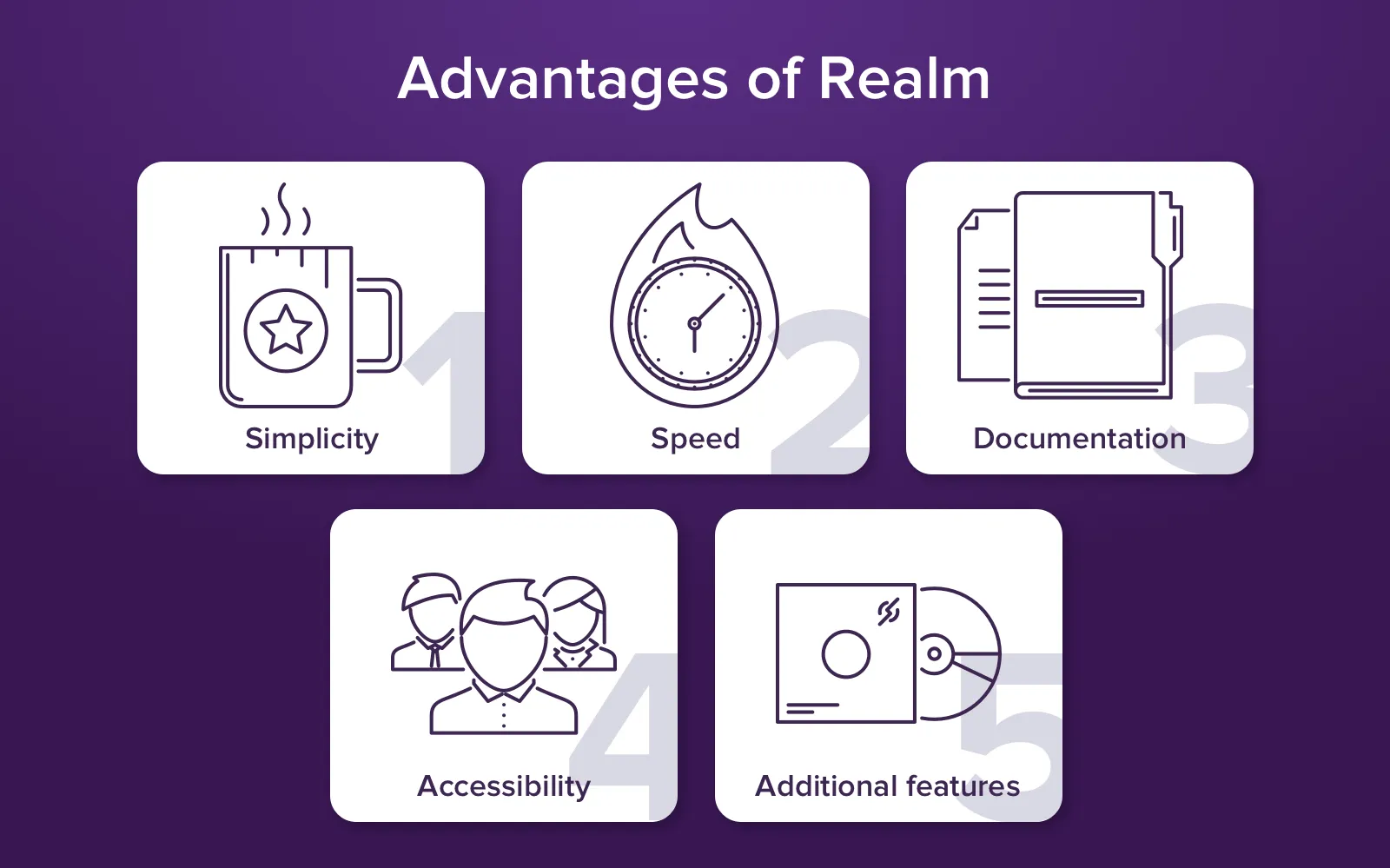
Pluses of Realm database for Android apps
1. Simplicity. Realm database is extremely simple compared to SQLite. It is much more laconic and requires you to write minimum code. For instance, to create a table using SQLite database in Android apps, you need to write such code:
private static final String SQL_CREATE_FAVOURITE =
"CREATE TABLE " + FavouritesDBContract.FavouritesEntry.TABLE_NAME + " (" +
FavouritesDBContract.FavouritesEntry._ID + INTEGER_TYPE + " PRIMARY KEY AUTOINCREMENT," +
FavouritesDBContract.FavouritesEntry.COLUMN_NAME_TITLE + TEXT_TYPE + COMMA_SEP +
FavouritesDBContract.FavouritesEntry.COLUMN_NAME_IMAGE_URL + TEXT_TYPE +
" )";
public void onCreate(SQLiteDatabase db) {
db.execSQL(SQL_CREATE_FAVOURITE);
}
The same task in Realm would require you to only inherit RealmObject.
public class Favourites extends RealmObject{
String title;
String imageLink;
@Nullable
ImageResponse image;
public Favourites(String title, String imageLink, @Nullable ImageResponse image) {
this.title = title;
this.imageLink = imageLink;
this.image = image;
}
}
Here's another example Realm vs SQLite. If you need to create a query to get a list of results, you have to write such code in SQLite:
@Override
public void getFavorites(int page, GetFavouritesCallBack callback)
List<Favourites> favourites = new ArrayList<>();
try {
Cursor cursor = mDb.query(
FavouritesDBContract.FavouritesEntry.TABLE_NAME, null, null, null, null, null, null);
if (cursor != null && cursor.getCount() > 0) {
while (cursor.moveToNext()) {
String title = cursor
.getString(cursor.getColumnIndexOrThrow(FavouritesDBContract.FavouritesEntry.COLUMN_NAME_TITLE));
String imagePath =
cursor.getString(cursor.getColumnIndexOrThrow(FavouritesDBContract.FavouritesEntry.COLUMN_NAME_IMAGE_URL));
Favourites favourite = new Favourites(title, imagePath, null);
favourites.add(favourite);
}
}
} catch (IllegalStateException e) {
callback.onDataNotAvailable();
}
}
And, of course, if we write the same code for Realm, we'll get something like this:
public List<Favourites> getFavorites() {
Realm realm = getRealm();
List<Favourites> favourites = realm.where(Favourites.class).findAll();
favourites = realm.copyFromRealm(favourites);
realm.close();
return favourites;
}
One of the brightest examples of the code complexity is a data migration issue. For instance, you use Realm database for Android project and you need to perform data migration. For this, you'll write:
public class FavouritesMigration implements RealmMigration {
@Override
public void migrate(DynamicRealm realm, long oldVersion, long newVersion) {
RealmSchema schema = realm.getSchema();
if (oldVersion == 1) {
schema.get("Favourites")
.removeField("image");
oldVersion++;
}
}
}
In config, you need to add:
private static final RealmConfiguration mConfig = new RealmConfiguration.Builder()
.schemaVersion(FAVOURITES_SCHEMA_VERSION)
.migration(new FavouritesMigration())
.build();
With SQLite, you'll have to create a similar table to the one you need to chance. Then you should copy all data from the old table, drop the old table and after that rename a new one. The main issue here is that you can't rename a table or a column, you cannot remove it as well. The same situation is with removing or adding constraints from or to a table.
As well as the code itself, we need to mention the ease of implementation while comparing SQLite and Realm databases. Realm is a very simple and intuitive system to work with. It's is a fully-functioning ORM platform. Which means you can save and read an object easily, and you don't need to think about internal collections. Everything works out-of-the-box.
Looking for some great free and open source libs for your Android app? Check our Git repository and read our report how we get in the top 3 on Github!
2. Speed. As we've noticed, Realm for Android development is really very fast. In some cases, it worked even faster than pure SQLite (we need to indicate that it was very simple SQL algorithm).
3. Documentation. It's always a great plus for any tool which we use. Realm is well documented and if there are any questions, you can always visit it's official website for more details.
4. Accessibility. Realm allows you to copy objects which remain accessible outside of the instance of Realm.
5. Additional features. As far as Realm is a much younger tool compared to SQLite, it can boast of many new features which are a great bonus for all developers. For instance, you can get a JSON support, encryption support and fluent API while using Realm in Android projects. What is more, there's a data change notifications feature available in Realm.
No wonder that so many people choose Realm. It seems way much easier than SQLite. Yet, we forget to tell you about Room which was released recently and gave a second life to SQLite database.
What is Room? Is it better than Realm?
Room is a library which was specifically designed by Google to smooth out all the weaknesses of SQLite. It provides an abstraction layer over SQLite, making the whole system much easier and effective for a developer to work with. Google developers managed not only to catch Room up but even outrun Realm in some cases. Of course, we needed to test it too. Here are the results of our investigation:
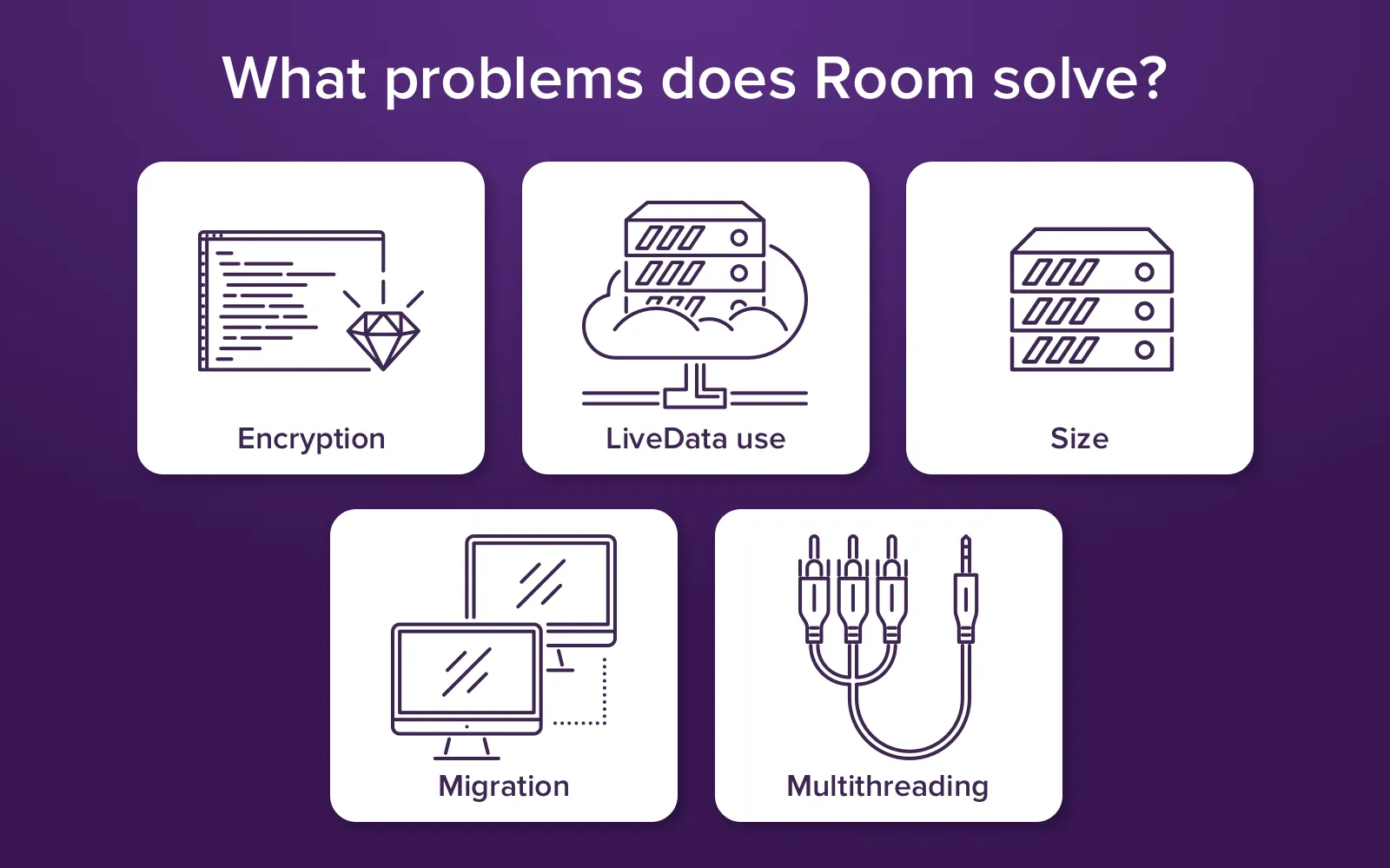
How Room makes SQLite better
Encryption
There's always a problem with this feature in SQLite, that's why Google developers added it to Room which helps this combo to compete with Realm DB
LiveData
It is easy to use LiveData in a few steps with the help of Room. No more problems and delays!
Still can't choose the best testing tool for your Android project? Here's a comprehensive comparison of the most effective testing frameworks from Cleveroad!
Size
Room is really very small, only 50 KB while the size of Realm DB can reach up to 3 or even 4 MB.
Data migration
This is probably one of the most pleasant things that we were so happy to see in Room. With this tool, data migration is not a problem anymore.
Multithreading
Some developers claim that they faced different multithreading issue working with Realm. Room doesn't have this problem at all.
Final thought
Just like with all other tools, every developer chooses which database to use for Android apps after some works and bumps, of course. The simplicity of Realm may give an impression that it's the best database for Android app development. Realm is easy to begin with, it is modern and has everything you need for a small and midsize project. It's a great turnkey solution which works straightforwardly without additional settings.
Yet, if development is not just a hobby for you, sooner or later you'll understand that SQLite and Room fit big and complex projects much better. You'll get acquainted with them learn how to love them. As for us, we use both solutions and choose the best database for Android apps according to the specification of a project and it's future architecture. If you need more information on the topic just write us and tell us more about your app idea!
Do you want to get more interesting articles from our custom Android app development company? Then subscribe to our blog and get tons of useful tips from the professionals!
Realm was designed specifically for mobile platforms. It has to make some trade-offs that boost it's performance. While SQLite is a Generic solution that was ported on mobile platforms.
There's no one-size-fits-all answer to this question. The reason is that different databases may suit better for different tasks. There's no unified database solution. Here, at Cleveroad, we use various databases and choose the best according to the specifications of the project.
If not get into details of project specifications, and consider the database features Realm may be the best solution for Android development. The simplicity of Realm may give the impression that it's the best database for Android app development. Realm is easy to begin with. It's modern and has everything you need for a small and midsize project. It's a great turnkey solution which works straightforwardly without additional settings.
A database is developed to store and access information. Most of apps collect a lot of data to function properly. If your app is simple and doesn't require a tons of data to be stored, then you can skip the database stage. However, it's better to discuss database specifications with software development company.
SQLite is used when you can't access a "real" database, but you still need the power of the relational database. The first field that comes to mind is IoT. It's hard to implement postgre or MySQL into small devices, so SQLite comes to help. It can be used in browsers because you can't know for sure whether users have MySQL installed on their PCs.
Realm is an object store database. It stores your data in a schema. However, it's not a relational database. It stores your data directly into the file.
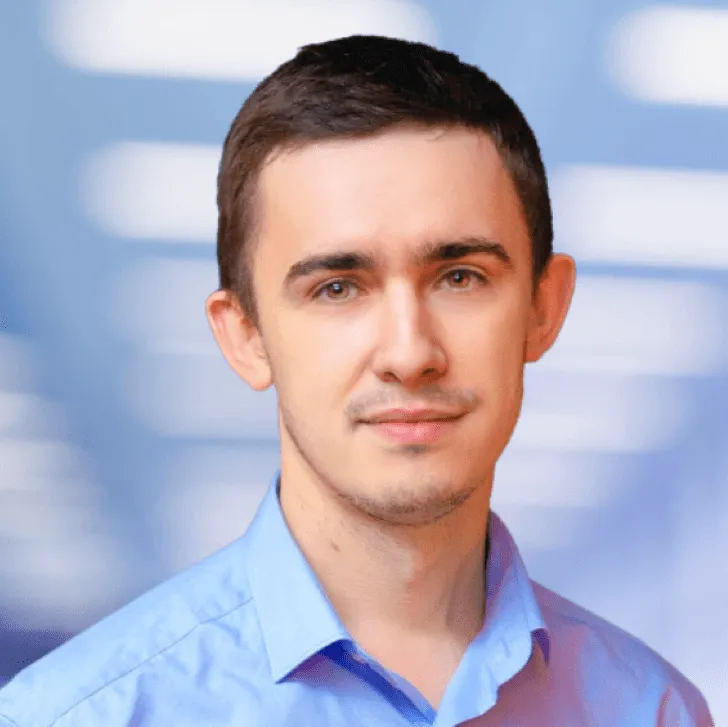
Evgeniy Altynpara is a CTO and member of the Forbes Councils’ community of tech professionals. He is an expert in software development and technological entrepreneurship and has 10+years of experience in digital transformation consulting in Healthcare, FinTech, Supply Chain and Logistics
Give us your impressions about this article
Give us your impressions about this article